- Web development
Moving DOM Elements Between Media Query Breakpoints
In this article, you will find a code snippet that moves HTML elements within the DOM based on media query breakpoints.
When implementing client web page designs, I sometimes encounter situations where an element needs to be placed in a completely different location depending on the media query breakpoints. If this issue cannot be resolved using only CSS, I need to change the element's position in the DOM.
To address this, I created a reusable code snippet, which you can find on Codepen. To test its behavior, simply resize the Codepen's browser window. When the window size reaches 1024px, the "Hello World" text will move between the red and blue rectangles.
Here is an explanation of key aspects
In your HTML, define a source element that encapsulates the elements to be moved within the DOM. For this source element, also specify a breakpoint in pixels at which the element should be moved.
<div data-moveelem-source="<codename>" data-moveelem-breakpoint="<breakpoint>">
<Elements that should be moved>
</div>
Additionally, define a target element in your HTML to which the moving elements will be relocated.
<div data-moveelem-target="<codename>"></div>
Below is an example with real values.
<div data-moveelem-source="hello-world" data-moveelem-breakpoint="1024">
<span>Hello world</span>
</div>
<div data-moveelem-target="hello-world"></div>
Finally, there is a script that performs the move functionality.
(() => {
const move = (sourceNode, targetTarget) => {
// Get all child elements of the source element
const sourceNodeChildren = Array.from(sourceNode.childNodes);
// And append the to the target element
for (let i = 0; i < sourceNodeChildren.length; i++) {
targetTarget.appendChild(sourceNodeChildren[i]);
}
};
// Listening to breakpoint and performing the move on breaktpoint hit
const listenMatchMedia = (sourceNode, targetTarget, breakpoint) => {
const mediaQuery = window.matchMedia(`(min-width: ${breakpoint}px)`);
const handleChange = (e) => {
if (e.matches) {
move(targetTarget, sourceNode);
} else {
move(sourceNode, targetTarget);
}
};
mediaQuery.addEventListener('change', handleChange);
handleChange(mediaQuery);
};
// Main function
const moveElems = () => {
// Get all source elements
const moveElemSourceNodes = document.querySelectorAll('[data-moveelem-source]');
// For all source elements
for (let i = 0; i < moveElemSourceNodes.length; i++) {
// Get breaktpoint number in px
const breakpoint = parseInt(moveElemSourceNodes[i].getAttribute('data-moveelem-breakpoint'), 10);
// Get codename that should be that same for source and target elements
const moveElemSourceCodename = moveElemSourceNodes[i].getAttribute('data-moveelem-source');
if (!moveElemSourceCodename || Number.isNaN(breakpoint)) continue;
// Get appropriate target element
const moveElemTargetNode = document.querySelector(`[data-moveelem-target="${moveElemSourceCodename}"]`);
if (!moveElemTargetNode) continue;
// When breakpoint is hit, move the elements
listenMatchMedia(moveElemSourceNodes[i], moveElemTargetNode, breakpoint);
}
};
moveElems();
})();
About the author
Milan Lund is a Full-Stack Web Developer, Solution Architect, and Consultant for Xperience by Kentico projects, working as both a freelancer and a contractor. He specializes in building and maintaining websites using Xperience by Kentico. Milan writes articles based on his project experiences to assist both his future self and other developers.
Find out more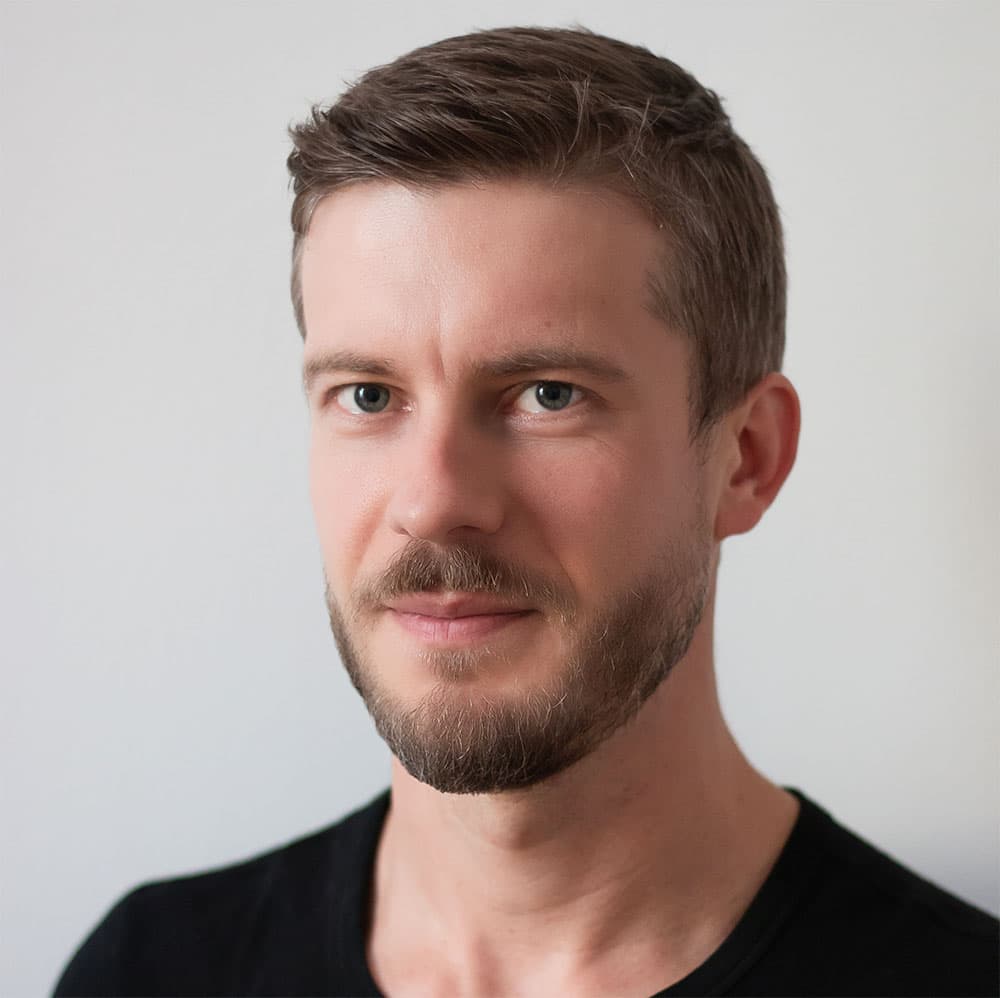